|
การอัพโหลดและดาวน์โหลดไฟล์ภาพด้วย WinSCP กับ Free Hosting - wwwServ00.com 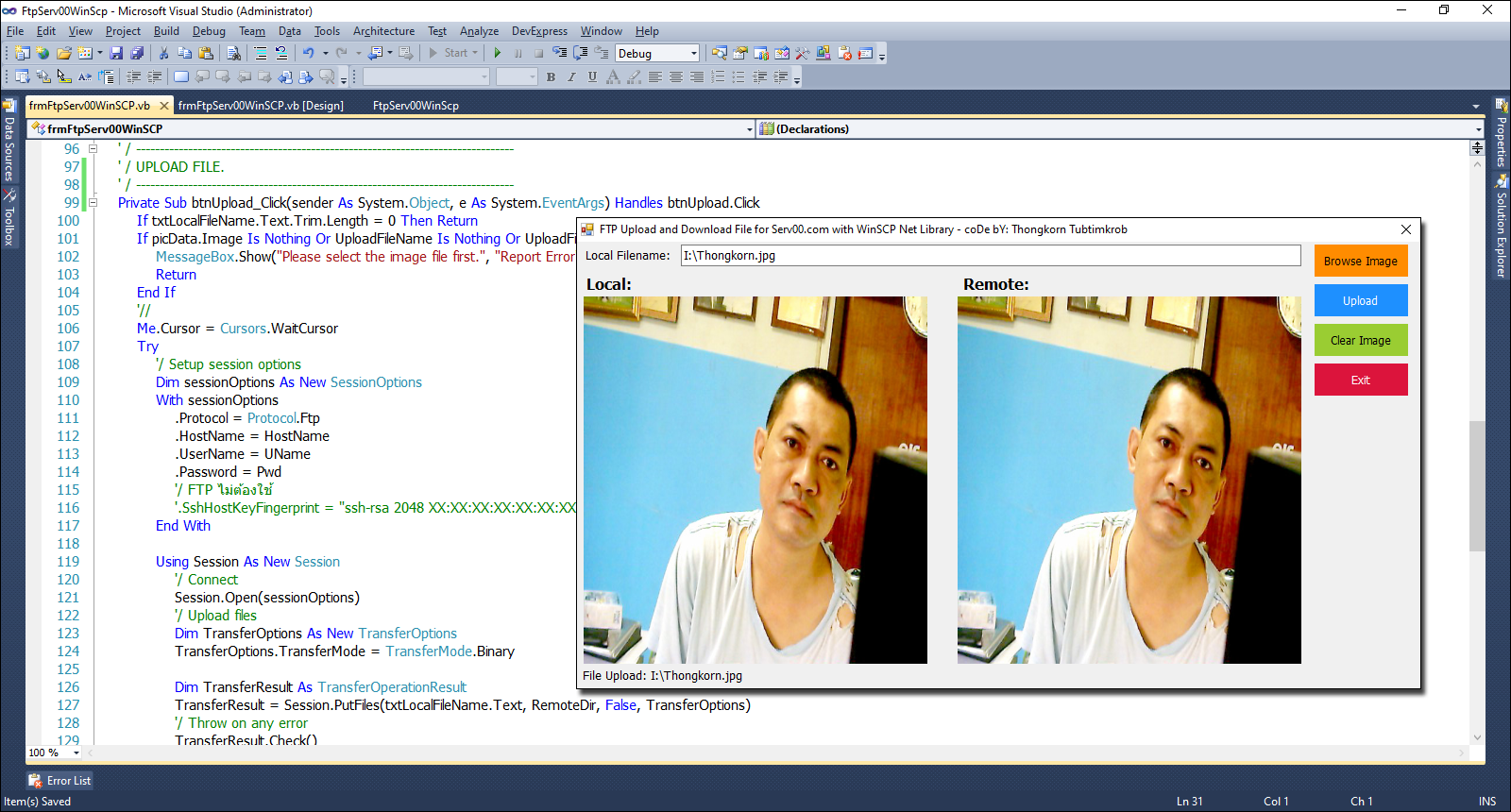
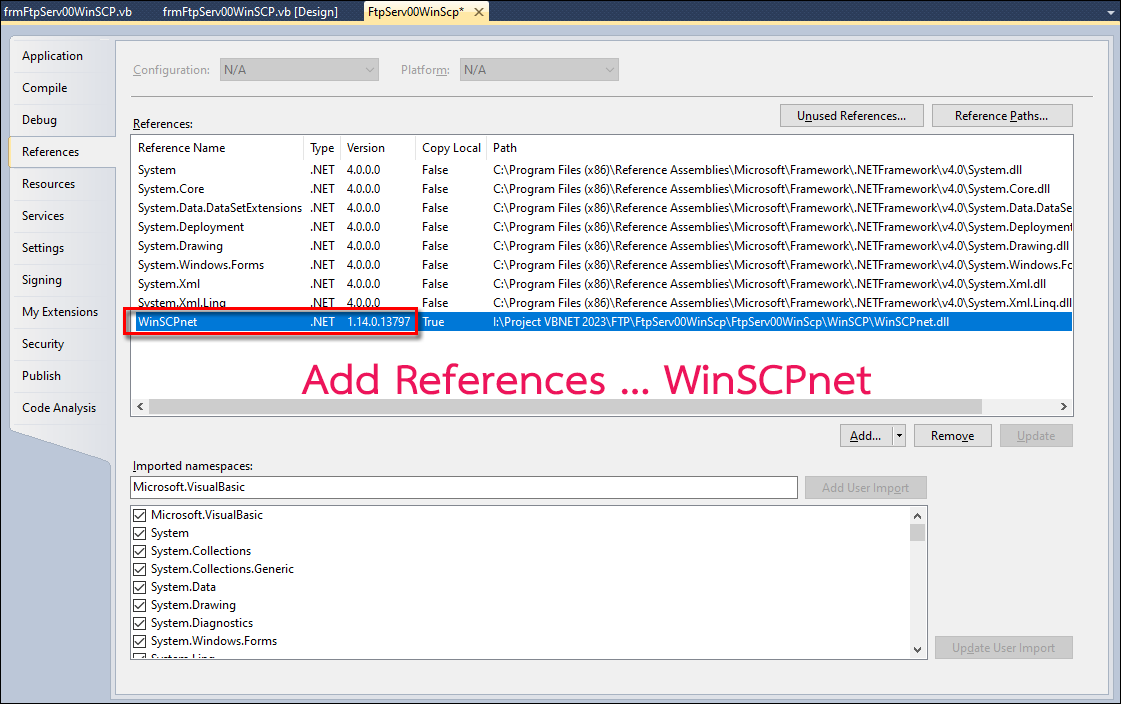
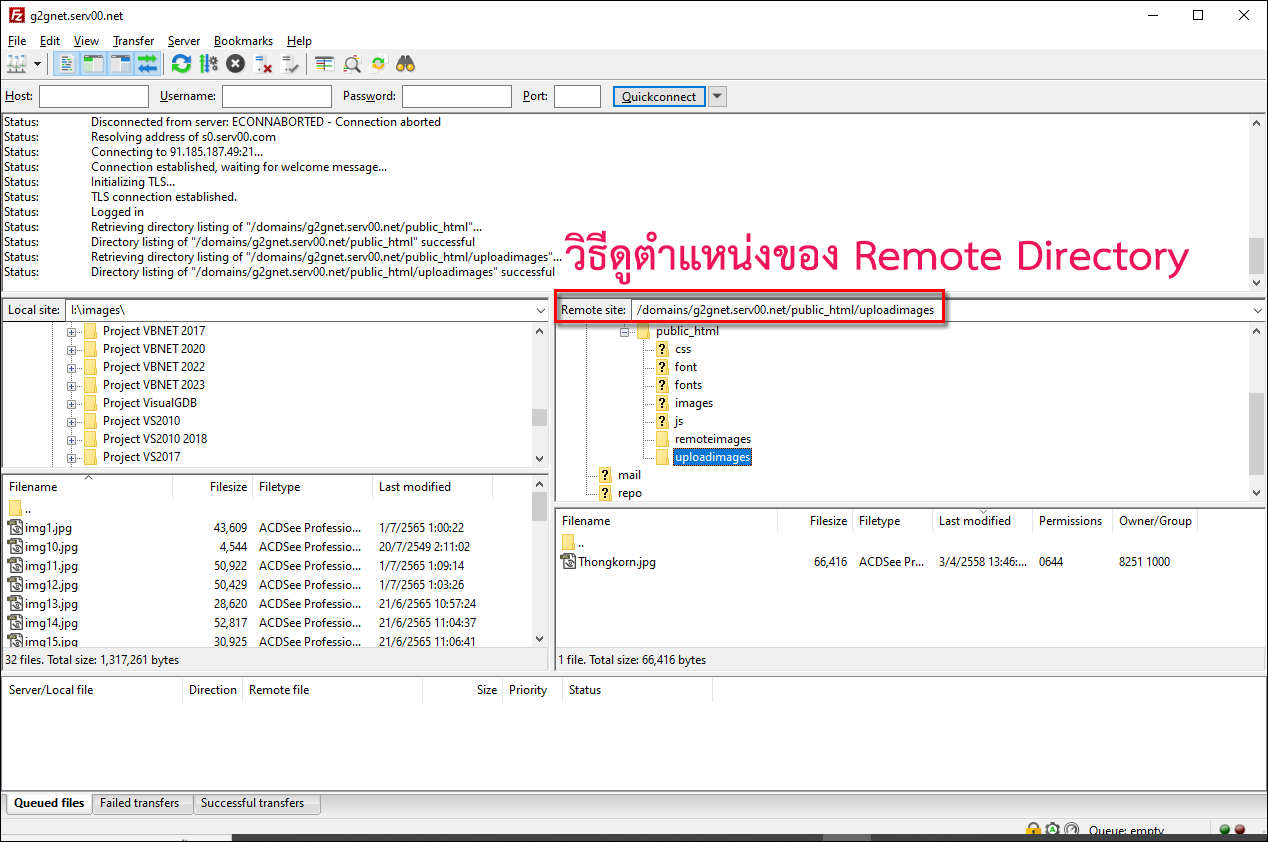
มาดูโค้ดฉบับเต็มกันเถอะ ...
- Imports System.IO
- Imports System.Net
- Imports WinSCP
- Public Class frmFtpServ00WinSCP
- ' / --------------------------------------------------------------------------------
- '// ชื่อไฟล์+นามสกุล เพื่อทำการ Upload ไปยัง Hosting
- Dim UploadFileName As String = ""
- '// Default path.
- Dim PicturePath As String = MyPath(Application.StartupPath) & "Images"
- ' / --------------------------------------------------------------------------------
- '// สำหรับ HOSTING
- '// ตำแหน่งของไฟล์ภาพบน Hosting เพื่อนำมาแสดงผล
- '// แก้ไข SUB DOMAIN และชื่อ UPLOADDIRECTORY ที่ต้องการอัพโหลดด้วยล่ะกัน
- Dim MyURL As String = "https://SUBDOMAIN.serv00.net/UPLOADDIRECTORY/"
- '// ตำแหน่งในการรีโมทเพื่อที่จะอัพโหลดไฟล์ภาพ (FTP) ...
- Dim RemoteDir As String = "domains/SUBDOMAIN.serv00.net/public_html/UPLOADDIRECTORY/"
- '// Login เข้าสู่ระบบ Hosting ...
- '// FTP Hostname ต้องดูจากตอนที่ Add Account for FTP เพราะอาจจะไม่เหมือนกับตัวอย่าง
- Dim HostName As String = "s0.serv00.com"
- Dim UName As String = "USERNAME"
- Dim Pwd As String = "PASSWORD"
- ' / --------------------------------------------------------------------------------
- ' / S T A R T ... H E R E
- ' / --------------------------------------------------------------------------------
- Private Sub frmFtpServ00WinSCP_Load(sender As System.Object, e As System.EventArgs) Handles MyBase.Load
- txtLocalFileName.Text = ""
- '/ Local image file.
- picData.Image = Image.FromFile(PicturePath + "NoImage.gif")
- picURL.Image = Image.FromFile(PicturePath + "NoImage.gif")
- Label1.Text = "File Upload: "
- End Sub
- ' / --------------------------------------------------------------------------------
- ' / เลือกรูปภาพในการ Upload.
- ' / --------------------------------------------------------------------------------
- Private Sub btnBrowse_Click(sender As System.Object, e As System.EventArgs) Handles btnBrowse.Click
- Dim dlgImage As OpenFileDialog = New OpenFileDialog()
- ' / Open File Dialog
- With dlgImage
- '.InitialDirectory = PicturePath
- .Title = "เลือกภาพ"
- .Filter = "รูปแบบภาพ (*.jpg;*.png;*.gif;*.bmp)|*.jpg;*.png;*.gif;*.bmp"
- .FilterIndex = 1
- .RestoreDirectory = True
- End With
- ' Select OK after Browse ...
- If dlgImage.ShowDialog() = DialogResult.OK Then
- ' Get file size
- Dim info As New FileInfo(dlgImage.FileName)
- If (info.Length / 1024) > 1024 Then
- MessageBox.Show("ไฟล์ภาพที่คุณเลือกมีขนาด " & Format((info.Length / 1024), "#,##0") & " KB. ซึ่งมีขนาดใหญ่เกินกว่า 1,024 KB.", "รายงานสถานะ", MessageBoxButtons.OK, MessageBoxIcon.Warning)
- Exit Sub
- End If
- '//
- txtLocalFileName.Text = dlgImage.FileName
- '// Put the current image file into PictureBox Control
- picData.Image = Image.FromFile(dlgImage.FileName)
- '// เอาเฉพาะชื่อไฟล์และนามสกุลภาพ (Filename + Extension) เช่น thongkorn.png
- UploadFileName = dlgImage.SafeFileName
- Label1.Text = "File Upload: " & txtLocalFileName.Text
- End If
- End Sub
- ' / --------------------------------------------------------------------------------
- ' / UPLOAD FILE.
- ' / --------------------------------------------------------------------------------
- Private Sub btnUpload_Click(sender As System.Object, e As System.EventArgs) Handles btnUpload.Click
- If txtLocalFileName.Text.Trim.Length = 0 Then Return
- If picData.Image Is Nothing Or UploadFileName Is Nothing Or UploadFileName.Length = 0 Or UploadFileName = "" Then
- MessageBox.Show("Please select the image file first.", "Report Error", MessageBoxButtons.OK, MessageBoxIcon.Warning)
- Return
- End If
- '//
- Me.Cursor = Cursors.WaitCursor
- Try
- '/ Setup session options
- Dim sessionOptions As New SessionOptions
- With sessionOptions
- .Protocol = Protocol.Ftp
- .HostName = HostName
- .UserName = UName
- .Password = Pwd
- '/ FTP ไม่ต้องใช้
- '.SshHostKeyFingerprint = "ssh-rsa 2048 XX:XX:XX:XX:XX:XX:XX:XX:XX:XX:XX:XX:XX:XX:XX:XX"
- End With
- '//
- Using Session As New Session
- '/ Connect with options.
- Session.Open(sessionOptions)
- '/ Upload files
- Dim TransferOptions As New TransferOptions
- TransferOptions.TransferMode = TransferMode.Binary
- Dim TransferResult As TransferOperationResult
- TransferResult = Session.PutFiles(txtLocalFileName.Text, RemoteDir, False, TransferOptions)
- '/ Throw on any error
- TransferResult.Check()
- '/ Show results.
- For Each Transfer In TransferResult.Transfers
- MessageBox.Show("Upload " & Transfer.FileName & " Successful.")
- Next
- End Using
- '// Download image file and show on PictureBox (picURL).
- Dim Req As WebRequest = WebRequest.Create(MyURL & UploadFileName)
- Dim Res As WebResponse = Req.GetResponse()
- Dim imgStream As Stream = Res.GetResponseStream()
- Dim imgPic As Image = Image.FromStream(imgStream)
- imgStream.Close()
- '/ Load File Stream into PictureBox.
- With picURL
- picURL.Image = imgPic
- .WaitOnLoad = True
- .SizeMode = PictureBoxSizeMode.StretchImage
- End With
- Catch ex As Exception
- MessageBox.Show(ex.Message)
- End Try
- Me.Cursor = Cursors.Default
- Label1.Text = "File Upload: " & txtLocalFileName.Text
- End Sub
- ' / --------------------------------------------------------------------------------
- ' / เคลียร์รูปภาพออกจาก PictureBox
- ' / --------------------------------------------------------------------------------
- Private Sub btnDeleteImg_Click(sender As System.Object, e As System.EventArgs) Handles btnDeleteImg.Click
- txtLocalFileName.Clear()
- '/ Local image file.
- picData.Image = Image.FromFile(PicturePath & "NoImage.gif")
- picURL.Image = Image.FromFile(PicturePath & "NoImage.gif")
- UploadFileName = ""
- Label1.Text = "File Upload: "
- End Sub
- Private Sub txtLocalFileName_KeyPress(sender As Object, e As System.Windows.Forms.KeyPressEventArgs) Handles txtLocalFileName.KeyPress
- '/ Lock keypress.
- e.Handled = True
- End Sub
- Private Sub frmFtpServ00WinSCP_FormClosed(sender As Object, e As System.Windows.Forms.FormClosedEventArgs) Handles Me.FormClosed
- Me.Dispose()
- GC.SuppressFinalize(Me)
- Application.Exit()
- End Sub
- Private Sub btnExit_Click(sender As System.Object, e As System.EventArgs) Handles btnExit.Click
- Me.Close()
- End Sub
- #Region "FUNCTION"
- ' / --------------------------------------------------------------------------------
- ' / Get my project path
- ' / AppPath = C:\My Project\bin\debug
- ' / Replace "\bin\debug" with ""
- ' / Return : C:\My Project\
- Function MyPath(ByVal AppPath As String) As String
- '/ Return Value
- MyPath = AppPath.ToLower.Replace("\bin\debug", "").Replace("\bin\release", "").Replace("\bin\x86\debug", "").Replace("\bin\x86\release", "")
- '// If not found folder then put the \ (BackSlash) at the end.
- If Microsoft.VisualBasic.Right(MyPath, 1) <> Chr(92) Then MyPath = MyPath & Chr(92)
- End Function
- #End Region
- End Class
คัดลอกไปที่คลิปบอร์ด
ดาวน์โหลดโค้ดต้นฉบับ VB.NET (2010) ได้ที่นี่ ...
|
ขออภัย! โพสต์นี้มีไฟล์แนบหรือรูปภาพที่ไม่ได้รับอนุญาตให้คุณเข้าถึง
คุณจำเป็นต้อง ลงชื่อเข้าใช้ เพื่อดาวน์โหลดหรือดูไฟล์แนบนี้ คุณยังไม่มีบัญชีใช่ไหม? ลงทะเบียน
x
|