|
การใช้งาน ListView ของ MaterialSkin2 ซึ่งจะใช้งานได้สำหรับ .NET Framework 4.5 ขึ้นไป ...
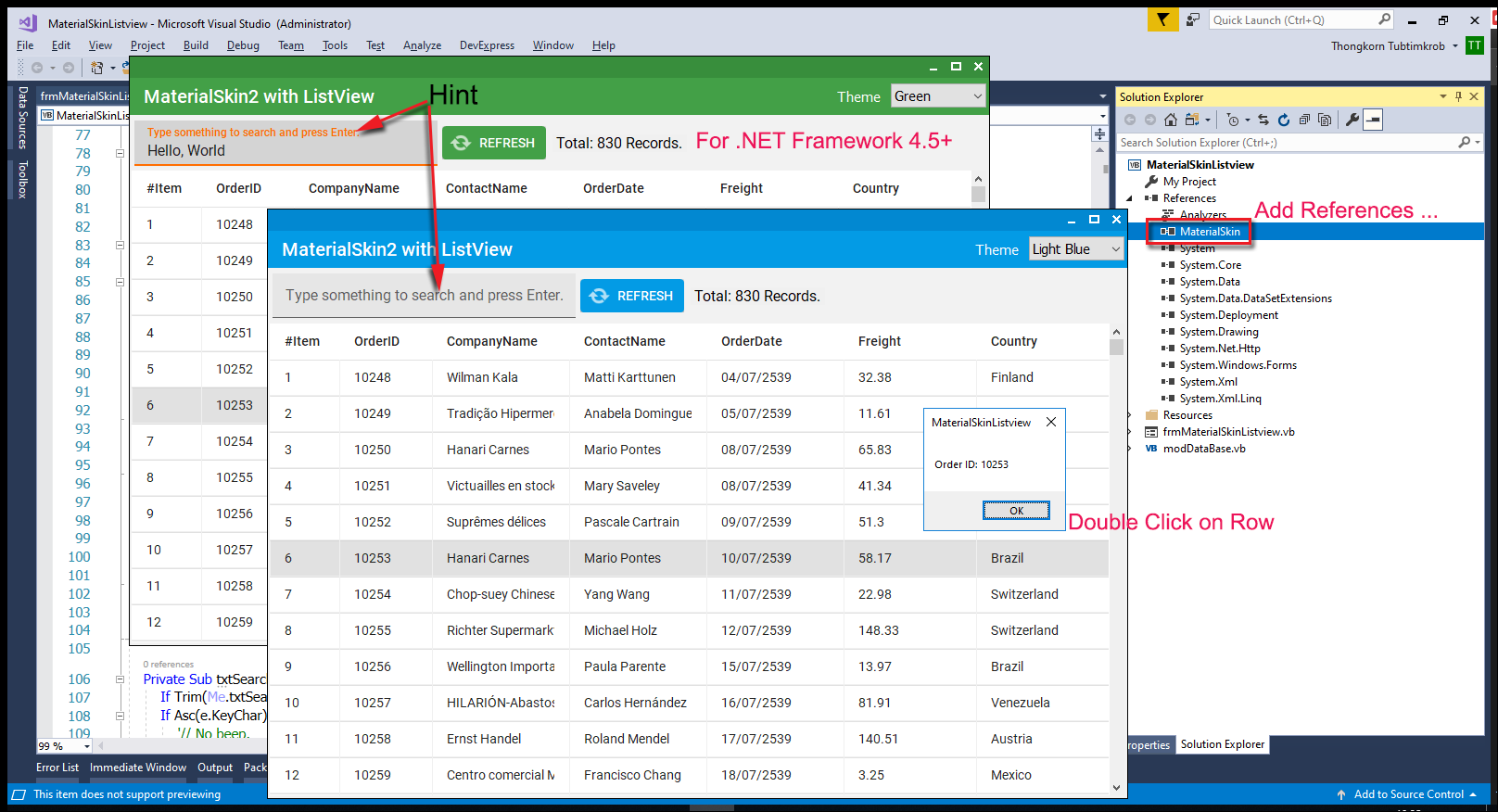
ดาวน์โหลดโค้ดต้นฉบับ Visual Basic .NET (2017) ได้ที่นี่ ...
มาดูโค้ดกันเถอะ ...
- '// Download packages.
- '// https://www.nuget.org/packages/MaterialSkin.2/
- Imports System.Data.OleDb
- Imports MaterialSkin
- Imports MaterialSkin.Controls
- Public Class frmMaterialSkinListview
- Private Sub frmMaterialSkinListview_Load(sender As Object, e As EventArgs) Handles MyBase.Load
- If Not ConnectDataBase() Then
- MessageBox.Show("Can't connect Northwind.accdb MS Access DataBase.", "Report Status", MessageBoxButtons.OK, MessageBoxIcon.Exclamation)
- Application.Exit()
- End If
- With cmbColorTheme
- .Items.Add("Orange")
- .Items.Add("Green")
- .Items.Add("Light Blue")
- .Items.Add("Cyan")
- .Items.Add("Gray")
- End With
- cmbColorTheme.SelectedIndex = 1
- lblRecordCount.Text = ""
- '// MaterialTextBox Properties. (Which you can customize at Design Time.)
- With txtSearch
- .Hint = "Type something to search and press Enter."
- .UseTallSize = True
- .UseAccent = True
- .Anchor = AnchorStyles.Left + AnchorStyles.Right + AnchorStyles.Top + AnchorStyles.Bottom
- End With
- '// Show all data.
- Call RetrieveData(False)
- End Sub
- ' / --------------------------------------------------------------------------------
- ' / Collect all searches, come in the same place.
- ' / blnSearch = True, Show that the search results.
- ' / blnSearch is set default to False, for show all records.
- Sub RetrieveData(Optional ByVal blnSearch As Boolean = False)
- strSQL =
- " SELECT Orders.OrderID, Customers.CompanyName, Customers.ContactName, Customers.Address, Customers.City, " &
- " Customers.Region, Customers.PostalCode, Customers.Country, Orders.OrderDate, Orders.Freight, Orders.ShipName " &
- " FROM Customers INNER JOIN Orders ON Customers.CustomerID = Orders.CustomerID "
- If blnSearch Then
- strSQL = strSQL &
- " WHERE " &
- " [OrderID] " & " Like '%" & txtSearch.Text & "%'" & " OR " &
- " [CompanyName] " & " Like '%" & txtSearch.Text & "%'" & " OR " &
- " [ContactName] " & " Like '%" & txtSearch.Text & "%'" & " OR " &
- " [Address] " & " Like '%" & txtSearch.Text & "%'" & " OR " &
- " [City] " & " Like '%" & txtSearch.Text & "%'" & " OR " &
- " [Region] " & " Like '%" & txtSearch.Text & "%'" & " OR " &
- " [PostalCode] " & " Like '%" & txtSearch.Text & "%'" & " OR " &
- " [Country] " & " Like '%" & txtSearch.Text & "%'" & " OR " &
- " [ShipName] " & " Like '%" & txtSearch.Text & "%'" &
- " ORDER BY OrderID "
- Else
- strSQL = strSQL & " ORDER BY OrderID "
- End If
- '//
- Call SetupListView()
- Try
- If Conn.State = ConnectionState.Closed Then Conn.Open()
- Cmd = New OleDb.OleDbCommand(strSQL, Conn)
- DR = Cmd.ExecuteReader()
- Dim iRow As Integer = 1 '// Counter
- Do While DR.Read()
- Dim NewItem As New ListViewItem(iRow)
- With NewItem.SubItems
- .Add(DR.Item("OrderID").ToString)
- .Add(DR.Item("CompanyName").ToString)
- .Add(DR.Item("ContactName").ToString)
- .Add(Convert.ToDateTime(DR.Item("OrderDate").ToString))
- .Add(DR.Item("Freight").ToString)
- .Add(DR.Item("Country").ToString)
- End With
- lvwData.Items.Add(NewItem)
- iRow += 1
- Loop
- lblRecordCount.Text = "Total: " & Format(lvwData.Items.Count, "#,##0") & " Records."
- DR.Close()
- Cmd.Dispose()
- Catch ex As Exception
- MessageBox.Show(ex.Message)
- End Try
- txtSearch.Text = String.Empty
- txtSearch.Focus()
- End Sub
- Private Sub txtSearch_KeyPress(sender As Object, e As KeyPressEventArgs) Handles txtSearch.KeyPress
- If Trim(Me.txtSearch.Text) = "" Or Len(Trim(txtSearch.Text)) = 0 Then Exit Sub
- If Asc(e.KeyChar) = 13 Then
- '// No beep.
- e.Handled = True
- '/ Retrieve Data(True) means that it is searching for information which user want.
- Call RetrieveData(True)
- End If
- End Sub
- ' / ----------------------------------------------------------------------------------------
- ' / Initailize MaterialListView Control
- Sub SetupListView()
- '// Properties of MaterialListView.
- With lvwData
- .Clear()
- .View = View.Details
- .GridLines = True
- .FullRowSelect = True
- .HideSelection = False
- .MultiSelect = False
- .GridLines = True
- .HoverSelection = True
- .OwnerDraw = True
- .Font = New Font("Roboto", 22, FontStyle.Regular) '// Row height adjustment Use the font size instead.
- ' 1st Column Index = 0
- .Columns.Add("#Item", 75)
- .Columns.Add("OrderID", 100)
- .Columns.Add("CompanyName", lvwData.Width \ 6 - 5)
- .Columns.Add("ContactName", lvwData.Width \ 6 - 5)
- .Columns.Add("OrderDate", lvwData.Width \ 6 - 5)
- .Columns.Add("Freight", lvwData.Width \ 6 - 10)
- .Columns.Add("Country", lvwData.Width \ 6 - 10)
- End With
- End Sub
- '// Select Color Scheme
- Private Sub cmbColorTheme_SelectedIndexChanged(sender As System.Object, e As System.EventArgs) Handles cmbColorTheme.SelectedIndexChanged
- Select Case cmbColorTheme.SelectedIndex
- Case 0
- SkinManager.ColorScheme = New ColorScheme(Primary.Amber500, Primary.BlueGrey900, Primary.BlueGrey500, Accent.Red700, TextShade.WHITE)
- Case 1
- SkinManager.ColorScheme = New ColorScheme(Primary.Green600, Primary.Green700, Primary.Green200, Accent.Orange700, TextShade.WHITE)
- Case 2
- SkinManager.ColorScheme = New ColorScheme(Primary.LightBlue600, Primary.LightBlue700, Primary.Green200, Accent.Purple700, TextShade.WHITE)
- Case 3
- SkinManager.ColorScheme = New ColorScheme(Primary.Cyan500, Primary.Cyan700, Primary.Cyan100, Accent.Blue700, TextShade.WHITE)
- Case 4
- SkinManager.ColorScheme = New ColorScheme(Primary.Grey600, Primary.Grey700, Primary.Grey100, Accent.Teal700, TextShade.WHITE)
- End Select
- txtSearch.Focus()
- End Sub
- '// Doublick mouse event for show OrderID.
- Private Sub lvwData_DoubleClick(sender As Object, e As EventArgs) Handles lvwData.DoubleClick
- MsgBox("Order ID: " & lvwData.SelectedItems(0).SubItems(1).Text)
- End Sub
- Private Sub btnRefresh_Click(sender As Object, e As EventArgs) Handles btnRefresh.Click
- Call RetrieveData(False) '// Show all data.
- End Sub
- Private Sub frmMaterialSkinListview_Resize(sender As Object, e As EventArgs) Handles Me.Resize
- If lvwData.Items.Count = 0 Then Return
- With lvwData
- .Columns(0).Width = 75
- .Columns(1).Width = 100
- .Columns(2).Width = lvwData.Width \ 6 - 5
- .Columns(3).Width = lvwData.Width \ 6 - 5
- .Columns(4).Width = lvwData.Width \ 6 - 10
- .Columns(5).Width = lvwData.Width \ 6 - 10
- .Columns(6).Width = lvwData.Width \ 6 - 10
- End With
- End Sub
- Private Sub frmMaterialSkinListview_FormClosed(sender As Object, e As FormClosedEventArgs) Handles Me.FormClosed
- Me.Dispose()
- GC.SuppressFinalize(Me)
- Application.Exit()
- End Sub
- End Class
คัดลอกไปที่คลิปบอร์ด
|
|